Types of Subject in Angular
An RxJS Subject is a type of Observable that permits values to be broadcast/multicast to many Observers.
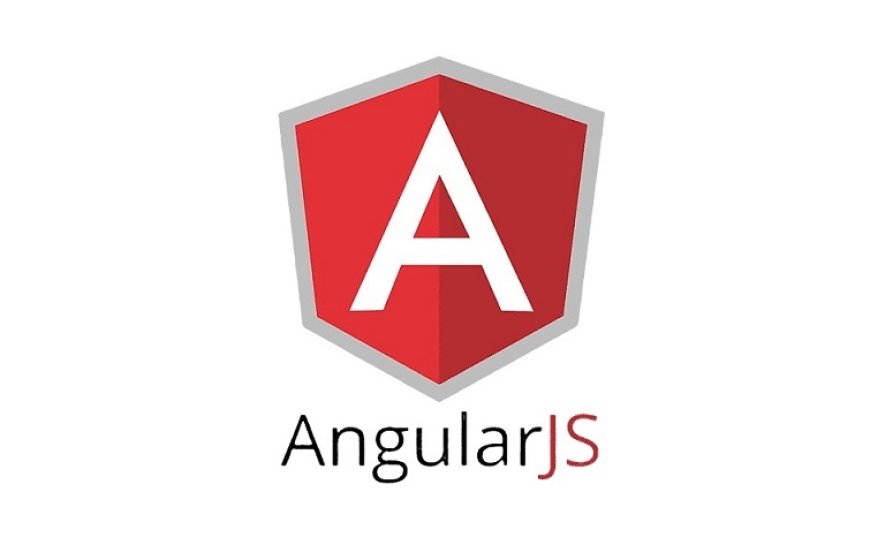
Subject Types
RxJS support types of subjects, which you can use for different scenarios:
1. Subject: a a basic implementation of a RxJS subject
A multicast observable that maintains a list of observers and notifies all of them when a new value is emitted using the next() method.
It does not have an initial value, so subscribers only receive values emitted after they subscribe.
Observables - Uni-Cast | Subject - Multi-Cast |
ngOnInit(): void {
let observable = new Observable<number>(ele =>
ele.next(Math.random()* 5))
//first observer/subscriber
observable.subscribe(result => {
const first_subscriber_observable = result;
console.log(result)
})
//second observer/subscriber
observable.subscribe(result => {
const second_subscriber_observable = result;
console.log(result)
})
//third observer/subscriber
observable.subscribe(result => {
const thrid_subscriber_observable = result;
console.log(result)
})
}
|
ngOnInit(): void {
let subject = new Subject<number>()
//first observer/subscriber
subject.subscribe(result => {
const first_subscriber_subject = result;
console.log(result)
})
//second observer/subscriber
subject.subscribe(result => {
const second_subscriber_subject = result;
console.log(result)
})
//third observer/subscriber
subject.subscribe(result => {
const third_subscriber_subject = result;
console.log(result)
})
subject.next(Math.random())
}
|
2. BehaviorSubject
Another type of subject in RxJS.
BehaviorSubject has an initial value that it will send immediately to any subscriber upon subscription, even if no values have been sent through the next() method yet.
Following the initial value's emission, it operates as a standard Subject and and notifies subscribers about new values emitted using next().
This comes in handy when you want to give new subscribers the last known value, such as the current state of an application or the latest data fetched from an API.
3. ReplaySubject
A subject that can buffer and replay a specific number of values to new subscribers. Replay Subjects keep a given number of historical values so that those values can be replayed to new subscribers.
The number of prior values that should be replayed to new subscribers is determined by the buffer size that you can define when creating a ReplaySubject.
This is helpful if you need to cache values for later usage or wish to give new subscribers a history of values.