Navigation between routes in Angular using RouterLink
Talking about routes is fine, but how does one really get to them? This can be accomplished with the routerLink directive.
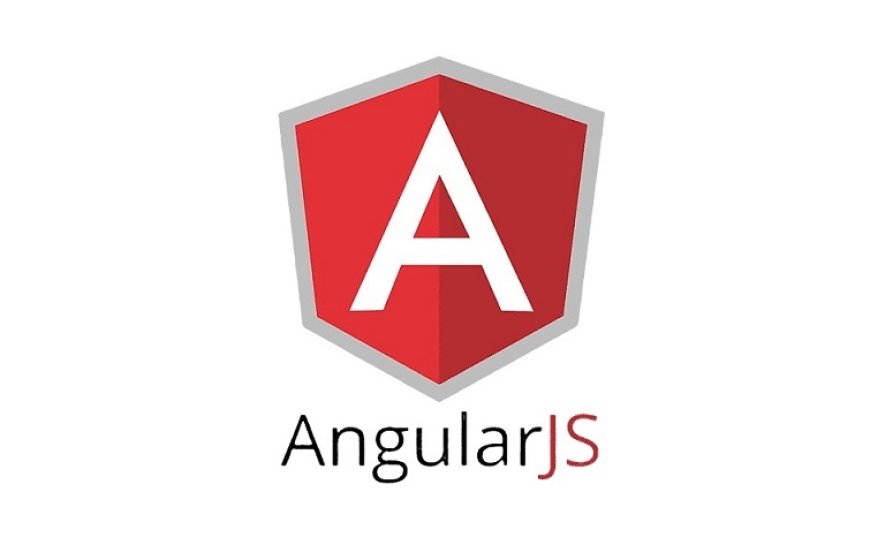
This article explains how to use RouterLink declarative navigation in Angular?
By clicking a link, button, or inputting a URL into the browser's address bar, the user can change the navigation or view. The data parameters from the previous view can be transferred to the new view with each view switch. The RouterLink directives let you link to specific parts of your app.
Add a navigation link (routerLink)
Note: To use routerLink directive in our Component, we need to add RouterModule or AppRoutingModule in @NgModule.imports of AppModule
Static Router Navigation
Either a string can be hardcoded directly into the template, as in the cases of the servers' or homes' routes above. Alternatively, we can pass it an expression. If so, we must provide it with an array that contains each of the several URL route segments we wish to visit; as in case of /users path above.
Dynamic Router Navigation
Another method for performing router navigation, is to use the router programmatic API. To accomplish this, all we have to do is inject the router into our component and use the navigate or navigateByUrl navigation methods.
Why Not Normal Anchor, Why RoutLink?
In case of normal anchor, when we are clicking any tab, it reloads entire application (refresh browser), instead of changing content and url only. Whereas RouteLink is a special directive. RouteLink instruct angular that the element on which routeLink is placed will server like a link but behave as a click
How Angular Prepare Routes
What happen if we specify routes without a leading /
If we provide links without leading / in app.component, It will works.
But if we provide link on inner page like servers.component.html, This gives you an error message
Reason: Without / it create a relative page, path relative to present working directory which is servers. In relative path, it always append the path specified in routeLink to end of your current path. With / it create absolute path from root directory, always get appended to the root domain.
Note:
./server is similar to server only (i.e. without any link)
../server - will go back one path above i.e. parent path