Subject in Angular
An RxJS Subject is a type of Observable that permits values to be broadcast/multicast to many Observers.
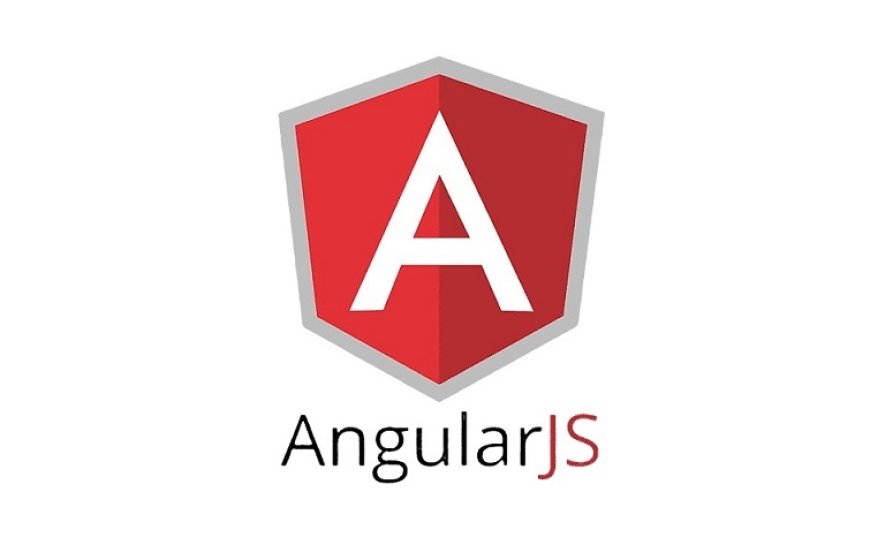
This Article helps to understand the concept of Subject in Angular
Subject can emit data, besides of its capability to be subscribed to
Where Subjects can use the next() function to send/emit events or data to their subscribers. On the other hand it uses subscribe() to subscribe to changes.
You may also cast Subjects to an Observable if you just want to show the ability to subscribe to changes and want to hide the Subject-like behaviour.
Difference between Observable and Subject
Observable and Subject are components of the Reactive Extensions for JavaScript (RxJS) framework, which Angular uses for event handling and asynchronous data stream management. They do, however, differ in a few significant ways.
- Observalbes are unicast, i.e., each subscribed observer/subscriber has its own execution of the observable, when you subscribe to an observable. Subjects, on the other hand, are multicast, i.e., they can maintain a list of observers/subscribers and notify all whenever a new value is emitted.
- Observables do not have an initial value. Observables can start emitting values only when an observer/subscriber is listening. Where a special type of Subject the BenaviorSubject on the other hand, can have its initial value. So when you subscribe to a BenaviorSubject, it will immediately emit the last value it received, if any, or the initial value, in case no value has been emitted yet to the subscriber.
- Observables are declarative, i.e. they do not emit value until a subscriber subscribes to them. Subjects are imperative, on the other hand i.e. they can produce values independently of whether there are any subscribers.
- Observables are generally used when you want to produce data in a lazy and declarative way, such as handling an HTTP requests, user input events, other async operations, etc. Subjects are commonly used where you want to multicast events or share data between multiple observer/subscriber as inter component communication or event broadcasting, etc.
Creating a Subject
Add More subscriber in Same Subject
Push Data into the subject: using next method