Extension methods in C#
Extension methods as the name implies is about extending the functionalities of the class.
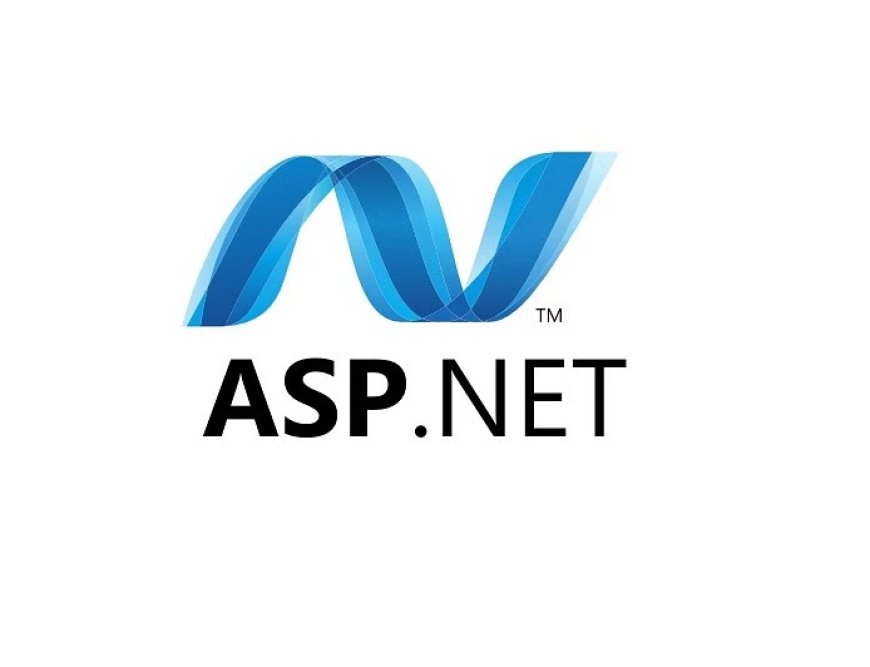
This Articles helps you to understand the concept of Extension methods in C#.
The Extension methods help you add a new method to the existing Class (or Type).
This is done without creating a new derived type or recompiling, or modifying the original type. Extension methods are only in scope when you explicitly import the namespace into your source code with a using directive.
Key Points of Extension methods
1. Class and Method must be defined as Static
2. First method is the type that is extended, Any other parameter will come after this.
Few Important about Extension method
- An extension method must be defined as a static method and must reside in a static class
- this keyword must be the first parameter of the extended method. This will actually inform the compiler that the method is an extension method.
- An extension method must be defined in the root of the namespace. It cannot be nested inside another class under the namespace
- The type that is being extended must be referenced in the extension method. Use the using statement to import the namespace.
- protected/private members of the type that is being extended will not be accessible from the Extension methods.
- You cannot override any method of the type you are extending.
- The Extension method has lower priority than the methods defined In the type. A similarly named method in an instance, always executed rather than the extended method. In fact, the extended method will never be called
- The Extension methods with the same name and signature may signature for the same class may be declared in multiple namespaces without causing compilation errors.
- Extension method cannot be used for adding a new properties, fields or events
Benefits of Extension method
1. Extension methods appear under the IntelliSense.
2. It makes the code more readable.
3. Extend the functionality of third party libraries where you don’t have access to the code. ( This may also break your code if the third party vendor changes the implementation of the library)