Understand Routing in Angular
Routing is only the act of flipping between pages. Because of routing, Angular users are able to create single-page apps with several views and switch among them. The application's features and state remain unaltered as users switch between these views.
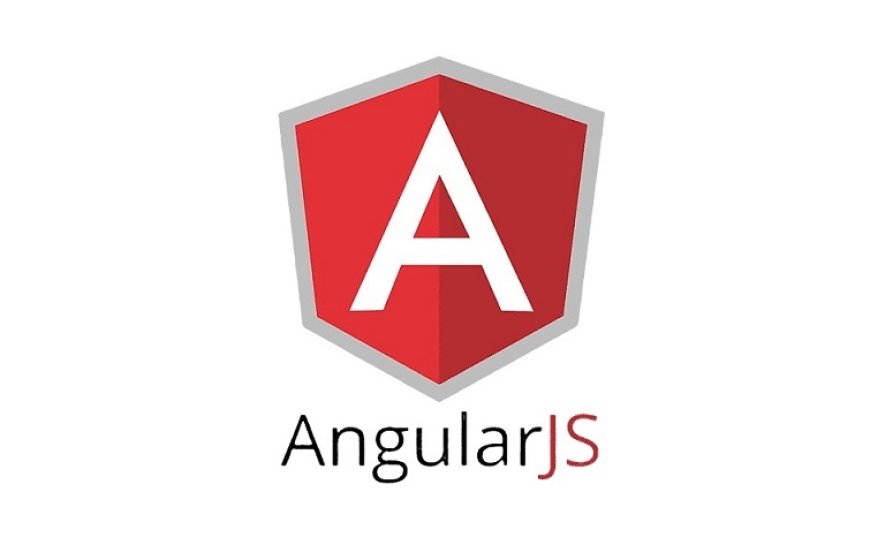
This Article helps you to Understand Angular Routing?
Angular Router
The @angular/router package contains Angular Router, a robust JavaScript router created and supported by the Angular core team. It offers a comprehensive routing library with the option of having numerous router outlets, various path matching algorithms, simple access to route parameters, and route guards to safeguard components from unwanted access.
The Angular platform's foundational element is the Angular router. Developers can use it to create Single Page Applications with many views and allow switching between them.
Routes and Paths
Routes are definitions (objects) made up of at least a component (or redirectTo path) property and a path.
The part of the URL known as the path indicates which particular view should be displayed.
The term "component" refers to the Angular component that requires a path to be attached to it.
The Router is able to direct the user to a certain view based on a route specification that we supply (through a static RouterModule.forRoot(routes) method).
Every Route connects a component to a URL path.
The path can be empty which denotes the default path of an application and it’s usually the start of the application.
Wildcard strings (**) are acceptable in the path. If the requested URL doesn't match any pathways for the defined routes, the router will choose this route. In the event that no match is discovered, this can be used to show a "Not Found" view or to reroute to a certain view.
An example of a route:
{ path: '', component: HomeComponent },
{ path: 'accounts', component: AccountListComponent},
If this route definition is provided to the Router configuration, the router will render AccountListComponent when the browser URL for the web application becomes /accounts.
Router Configuration
Simply writing down some routing settings should be our initial action. In this initial configuration, we're mapping specific URL paths to Angular components, which means that the component will be displayed if the path matches.
Bootstrapping the Router
We also need to include the router's directives and injectables into the Angular bootstrap system to complete the router setup. We accomplish this by importing the RouterModule into the application root module:
Why order is important for home and fallback routes
The concept of empty paths and wildcards are supported by the new component router, so we can set up an index route and a fallback route as follows:
import { NgModule } from "@angular/core";
import { Routes, RouterModule } from "@angular/router";
const appRoutes: Routes = [
{ path: "", component: HomeComponent },
{ path: "articles", component: ArticelsComponent },
{ path: "article/:id/:name", component: ArticleComponent },
{ path: "not-found", component: PageNotFoundComponent },
{ path: "**", redirectTo: "/not-found" },
];
@NgModule({
imports: [RouterModule.forRoot(appRoutes)],
exports: [RouterModule],
})
export class AppRoutingModule {}
We can see that the component Home would be mapped to the URL / and all other URLs to PageNotFoundComponent if the empty path setting were used. But this arrangement also has a disadvantage.